What Are TypeScript Decorators? 🤔
At the core, TypeScript decorators are a way to add metadata or behavior to a class, method, property, or parameter. They provide a powerful tool for customizing and extending the behavior of your code. Think of them as ‘annotations’ 🏷️ that allow you to modify your classes and their members.
Understanding the Basics
Creating Decorators:
Before we dive into practical examples, let’s understand how to create a decorator. A decorator is essentially a function prefixed with the @
symbol 💫. Here’s a simple example:
function log(target: any, propertyKey: string, descriptor: PropertyDescriptor) {
console.log(`Logging for ${propertyKey}`);
}
Applying Decorators:
To apply a decorator to a class or class member, you simply use the @
symbol followed by the decorator’s name:
class Example {
@log
someMethod() {
// Your method logic here
}
}
Common Use Cases 🚀
- Logging 🐞:Decorators like
log
above can be used to log method calls, making debugging a breeze. - Validation:Decorators can be applied to input parameters to validate their values, ensuring data integrity.
- Authentication:Secure your APIs by using decorators to check user permissions before executing a method.
- Dependency Injection:Use decorators to handle dependency injection, simplifying complex setups.
Advanced Decorator Features
Chaining Decorators ⛓️:
You can apply multiple decorators to the same class or member and control their execution order.
class Example {
@decorator1
@decorator2
someMethod() {
// Your method logic here
}
}
Parameterized Decorators ⏱️:
Parameterized decorators allow you to pass configuration options or parameters to the decorator function, which can modify its behavior based on those inputs. Here’s an example:
function logExecutionTime(logTime: boolean) {
return function (target: any, propertyKey: string, descriptor: PropertyDescriptor) {
const originalMethod = descriptor.value;
descriptor.value = function (...args: any[]) {
const startTime = performance.now();
const result = originalMethod.apply this, args);
if (logTime) {
const executionTime = performance.now() - startTime;
console.log(`Method ${propertyKey} took ${executionTime}ms to execute.`);
}
return result;
};
return descriptor;
};
}
class Example {
@logExecutionTime(true) // Pass true to log execution time
someMethod() {
// Your method logic here
for (let i = 0; i < 1000000; i++) {} // Simulate some work
}
}
const example = new Example();
example.someMethod(); // Method someMethod took XXXms to execute.
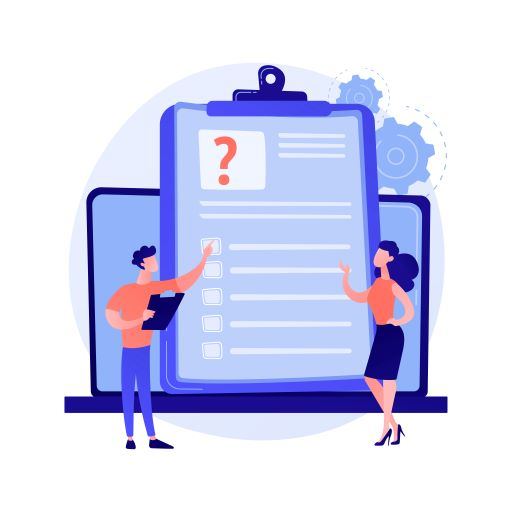
Best Practices 📋
- Keep It Simple:
Decorators should serve a single, clear purpose. Don’t overcomplicate your code with too many decorators on a single entity. - Document Your Code:
Add comments and documentation to explain what each decorator does and how to use it. - Test Rigorously:
Test your decorators thoroughly to ensure they behave as expected. - Stay Updated:
Decorator usage may change with TypeScript updates, so keep your TypeScript version up to date.
Conclusion
TypeScript decorators are a powerful tool for customizing and enhancing your code. They provide a way to add metadata and behavior to your classes, methods, and properties, making your code more readable and maintainable. By understanding the basics, common use cases, and best practices, you can harness the full potential of decorators in your TypeScript projects.
Remember, decorators are meant to simplify and improve your code, so use them wisely and make your codebase cleaner and more robust.
Happy coding! 🚀